Using Next.js for Better SEO: A Comprehensive Guide
Jason Chau
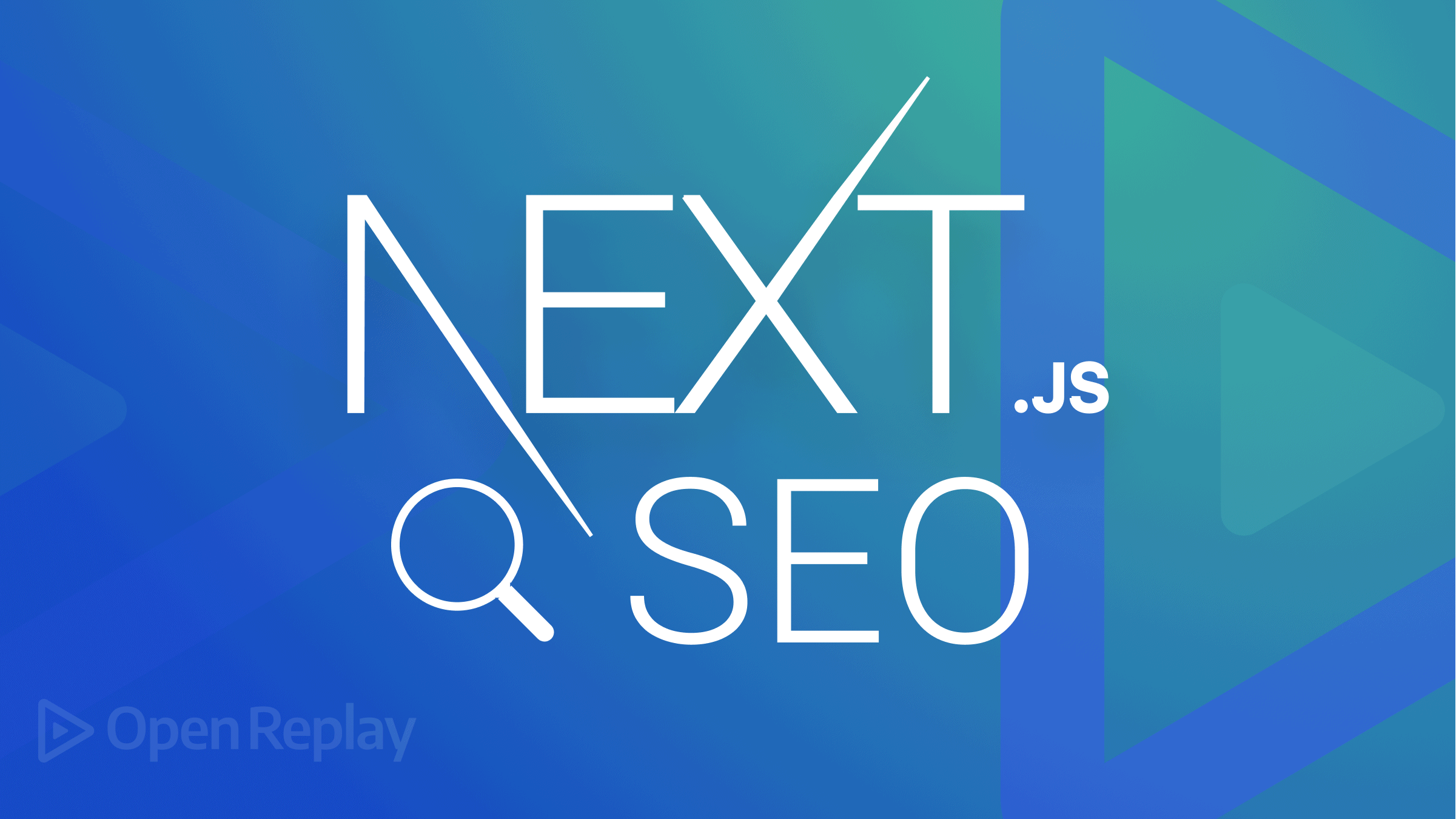
Table of Contents
Next.js is a powerful framework for building modern web applications, offering tools and features that significantly enhance Search Engine Optimization (SEO). By leveraging the app/ directory and its advanced rendering techniques, developers can create websites that are both performant and SEO-friendly. This article explores how to use the Next.js App Router, rendering methods (SSR, ISR, and client-side rendering), and features like generateStaticParams, generateMetadata, and revalidate to optimize your web application’s SEO.
Why SEO Matters in Modern Web Development
SEO is critical for increasing your website’s visibility in search engines. A well-optimized website:
• Improves discoverability.
• Enhances user experience with fast load times.
• Drives organic traffic.
Next.js excels at enabling SEO optimization by supporting various rendering techniques and dynamic features.
Next.js App Router and Its Role in SEO
The Next.js App Router, introduced in version 13, offers a new way to structure your application using the app/ directory. It simplifies server-side data fetching, dynamic routes, and metadata management, making SEO optimization easier and more integrated.
Key Features for SEO:
1. Dynamic Metadata Management: Using the generateMetadata function.
2. Improved Routing: Enables dynamic routes with generateStaticParams.
3. Integrated Data Fetching: Provides flexibility to use SSR, ISR, or client-side rendering.
Rendering Methods in Next.js
1. Server-Side Rendering (SSR)
SSR generates the HTML for a page on the server for each request. This ensures search engines crawl a fully-rendered page, making it SEO-friendly.
Use Case:
• Dynamic content that changes frequently (e.g., personalized user dashboards or frequently updated news pages).
Implementation:
In the app/ directory, you can define a fetch function directly inside your component to enable SSR:
export default async function Page() {
const data = await fetch('https://api.example.com/data', { cache: 'no-store' });
return <div>{data.title}</div>;
}
2. Incremental Static Regeneration (ISR)
ISR allows you to serve static pages while updating them incrementally. It combines the speed of static pages with the flexibility of SSR.
Use Case:
• Semi-static content like blogs or product pages that update periodically.
Implementation:
Use the revalidate property to define the interval (in seconds) for regenerating a page:
export async function getStaticProps() {
const data = await fetch('https://api.example.com/data');
return {
props: { data },
revalidate: 60, // Regenerate page every 60 seconds
};
}
3. Client-Side Rendering (CSR)
CSR loads a minimal HTML skeleton and uses JavaScript to fetch and render content on the client. While not ideal for SEO, it can complement SSR or ISR for non-critical sections of a page.
Use Case:
• Interactive dashboards or components that don’t need SEO prioritization.
Implementation:
Use a useEffect hook to fetch data client-side:
import { useEffect, useState } from 'react';
export default function Page() {
const [data, setData] = useState(null);
useEffect(() => {
fetch('https://api.example.com/data')
.then(res => res.json())
.then(data => setData(data));
}, []);
return <div>{data?.title}</div>;
}
Dynamic Features for SEO Optimization
1. generateStaticParams
generateStaticParams is used to pre-render dynamic routes at build time. This is particularly useful for pages like blogs or product details.
Example:
export async function generateStaticParams() {
const posts = await fetch('https://api.example.com/posts').then(res => res.json());
return posts.map(post => ({ slug: post.slug }));
}
In your dynamic route component:
export default function BlogPost({ params }) {
const { slug } = params;
// Fetch and render blog post based on slug
}
2. generateMetadata
generateMetadata allows you to dynamically define metadata for each page, improving SEO with accurate titles, descriptions, and Open Graph tags.
Example:
export async function generateMetadata({ params }) {
const post = await fetch(`https://api.example.com/posts/${params.slug}`).then(res => res.json());
return {
title: post.title,
description: post.excerpt,
openGraph: {
title: post.title,
description: post.excerpt,
images: post.image,
},
};
}
3. revalidate
The revalidate property is used with ISR to specify how often a page should be updated. This ensures your content is always fresh, boosting SEO.
Example:
export async function getStaticProps() {
const data = await fetch('https://api.example.com/data');
return {
props: { data },
revalidate: 10, // Regenerate the page every 10 seconds
};
}
Best Practices for Using Next.js for SEO
1. Use SSR or ISR for SEO-Critical Pages: Ensure important content like blogs, product pages, or landing pages is server-rendered.
2. Optimize Metadata: Always provide dynamic metadata using generateMetadata for personalized SEO.
3. Leverage Dynamic Routes: Use generateStaticParams for pre-rendering all possible routes.
4. Reduce JavaScript Usage: Keep critical content in SSR or ISR to minimize reliance on client-side rendering.
5. Set Up Revalidation: Use revalidate to balance freshness and performance for semi-static content.
Conclusion
Next.js provides powerful tools to build SEO-optimized web applications. By using the App Router, SSR, ISR, and features like generateStaticParams, generateMetadata, and revalidate, you can ensure your content is not only user-friendly but also highly discoverable by search engines.
For developers seeking better SEO performance, mastering these Next.js features is a crucial step forward.